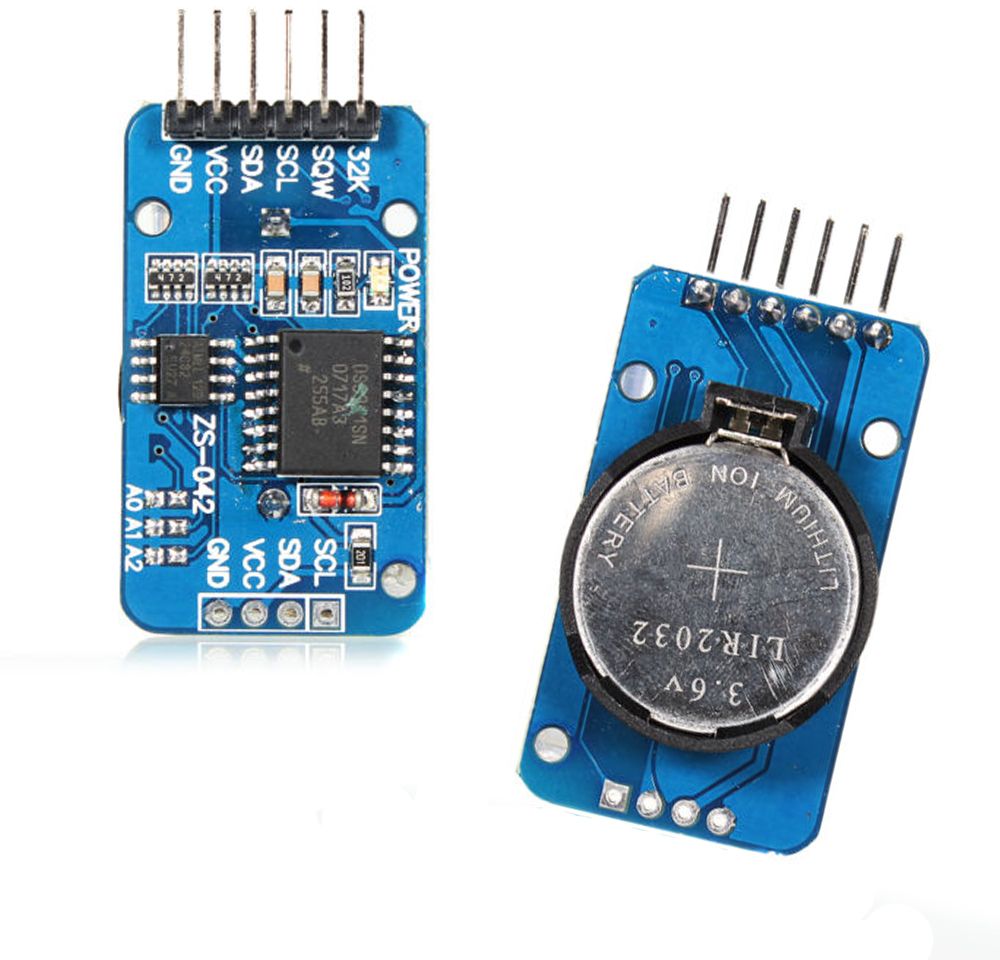
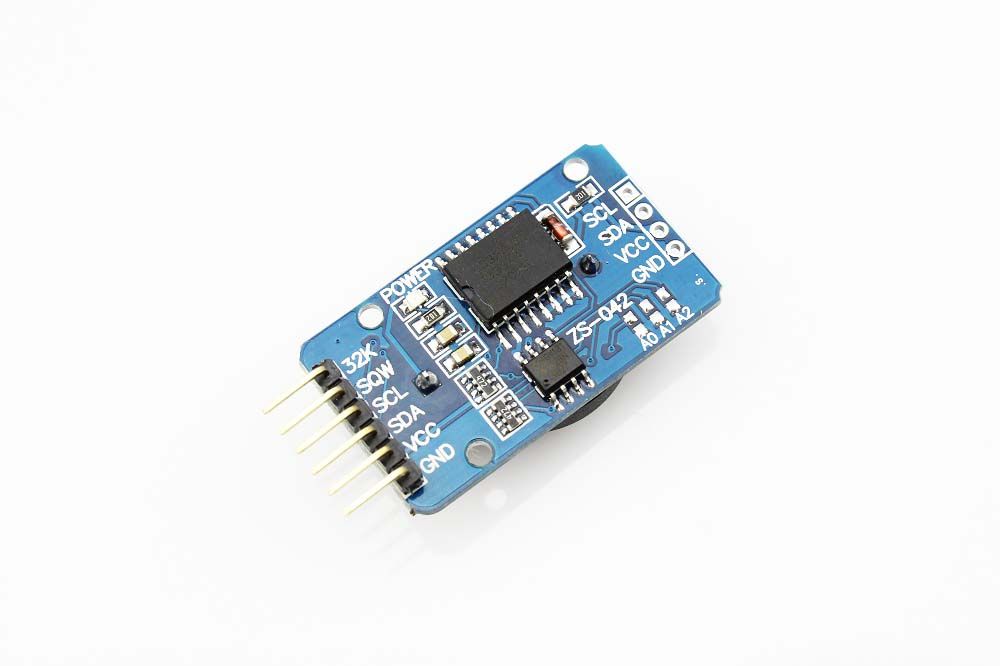
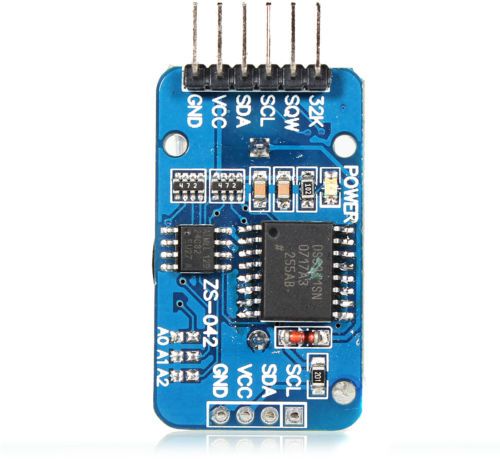
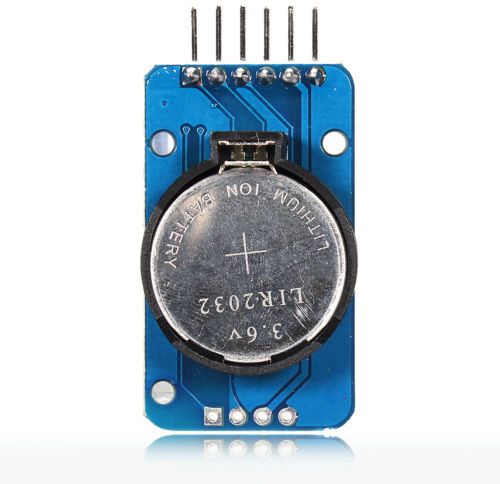
Description
- DS3231 is a low-cost real-time clock (RTC) that has an integrated temperature-compensated crystal oscillator (TCXO) and I2C working protocol. It also has a backup battery, which provides power when the main supply is cut off.
- It has a crystal resonator, which enhances the long-term accuracy of the device and reduces the piece-part count in a manufacturing line.
- It is available in a 16-pin, 300-millimeter SO package. This RTC module maintains seconds, minutes, hours, dates, months, and yearly information. It changes date and time at the end of the month automatically, including corrections for the leap year.
- This module operates in either a 24-hour or 12-hour format with an AM/PM indicator.
- It consists of a temperature-compensated voltage reference and comparator circuit, which monitors the status of Vcc to detect power failures. This circuit provides a reset output and automatically switches to a backup supply when necessary.
Â
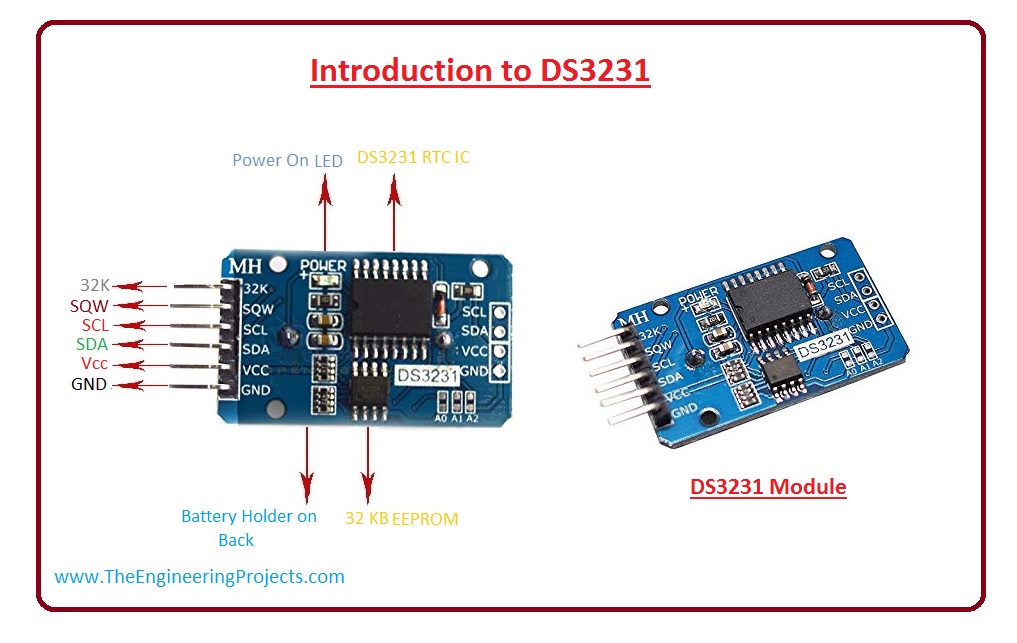
Package Includes
- 1 x DS3231 Real-Time Clock Module
Features
- The features of an electronic component can help you better understand the major functions associated with it. It will help you make a final decision before picking a device for your relevant project. Following are some features of DS3231.
- Its accuracy from 0°C to +40°C is ±2 ppm, and -40°C to +85°C is ±3.5 ppm.
- It is a low-power device. It has a battery backup for continuous timekeeping.
- Its temperature range for commercial use is 0°C to +70°C, and for industries, it is -40°C to +85°C.
- This real-time clock counts seconds, minutes, hours, days, dates, months, and years with leap-year compensation valid up to 2100.
- It has two times-a-day alarms. It can operate up to 400 kHz.
- It has a simple serial interface that can be connected to most microcontrollers. Its working protocol is I2C.
- Underwriters Laboratories (UL) is recognized.
Pinout
The DS3231 RTC module has 6 pins in total. The pinout is as follows:
32KÂ pin outputs a stable (temperature-compensated) and accurate reference clock.
INT/SQWÂ pin provides either an interrupt signal (due to alarm conditions) or a square-wave output at either 1Hz, 4kHz, 8kHz, or 32kHz.
SCLÂ is a serial clock pin for the I2C interface.
SDAÂ is a serial data pin for the I2C interface.
VCCÂ provides power to the module. You can connect it to a 3.3 to 5 volt power supply.
GNDÂ is the ground pin.
Wiring a DS3231 RTC module to an Arduino
Let’s connect the RTC to the Arduino.
Connections are straightforward. Begin by connecting the VCC pin to the Arduino’s 5V output and the GND pin to ground.
Now we are left with the pins that are used for I2C communication. Note that each Arduino board has different I2C pins that must be connected correctly. Â On Arduino boards with the R3 layout, the SDA (data line) and SCL (clock line) are on the pin headers close to the AREF pin. They are also referred to as A5 (SCL) and A4 (SDA).
The following table lists the pin connections:
DS3231 Module | Arduino | |
VCC | 5V | |
GND | GND | |
SCL | SCL or A5 | |
SDA | SDA or A4 |
The diagram below shows how to connect everything.
Installing uRTCLib Library
It takes a lot of effort to communicate with an RTC module. Fortunately, the uRTCLib library was created to hide all of the complexities, allowing us to issue simple commands to read the RTC data.
It is a simple yet powerful library that also supports time-of-day alarms and programming the SQW output, which is not supported by many RTC libraries.
To install the library, navigate to Sketch > Include Library > Manage Libraries… Wait for the Library Manager to download the library index and update the list of installed libraries.
Filter your search by entering ‘urtclib’. Look for uRTCLib by Naguissa. Click on that entry and then choose Install.

At the end of the tutorial, we’ve also included code for reading and writing the onboard 24C32 EEPROM. If you’re interested, you’ll need to install the uEEPROMLib library. Look for ‘ueepromlib‘ and install it as well.

Arduino Code – Reading Date, Time and Temperature
This is a simple sketch for setting/reading the date, time, and temperature from the DS3231 RTC module.
#include "Arduino.h"
#include "uRTCLib.h"
// uRTCLib rtc;
uRTCLib rtc(0x68);
char daysOfTheWeek[7][12] = {"Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"};
void setup() {
Serial.begin(9600);
delay(3000); // wait for console opening
URTCLIB_WIRE.begin();
// Comment out below line once you set the date & time.
// Following line sets the RTC with an explicit date & time
// for example to set January 13 2022 at 12:56 you would call:
rtc.set(0, 56, 12, 5, 13, 1, 22);
// rtc.set(second, minute, hour, dayOfWeek, dayOfMonth, month, year)
// set day of week (1=Sunday, 7=Saturday)
}
void loop() {
rtc.refresh();
Serial.print("Current Date & Time: ");
Serial.print(rtc.year());
Serial.print('/');
Serial.print(rtc.month());
Serial.print('/');
Serial.print(rtc.day());
Serial.print(" (");
Serial.print(daysOfTheWeek[rtc.dayOfWeek()-1]);
Serial.print(") ");
Serial.print(rtc.hour());
Serial.print(':');
Serial.print(rtc.minute());
Serial.print(':');
Serial.println(rtc.second());
Serial.print("Temperature: ");
Serial.print(rtc.temp() / 100);
Serial.print("\xC2\xB0"); //shows degrees character
Serial.println("C");
Serial.println();
delay(1000);
}
Here’s what the output looks like:

Code Explanation:
The sketch begins by including the Arduino.h and uRTCLib.h libraries for communicating with the module. We then create an uRTCLib library object and define the daysOfTheWeek
 2D character array to store the days information.
To interact with the RTC module, we use the following functions in the setup and loop sections of the code:
begin()Â function ensures that the RTC module is properly initialized.
set(ss, mm, hh, day, dd, mm, yy)Â function sets the RTC to an explicit date and time. For example, to set January 13, 2022 at 12:56, you would call:Â rtc.set(0, 56, 12, 5, 13, 1, 22);
refresh()Â function refreshes data from the HW RTC.
year()Â function returns the current year.
month()Â function returns the current month.
day()Â function returns the current day.
dayOfWeek()Â function returns the current day of the week (1 to 7). This function is typically used as an index for a 2D character array that stores information about the days.
hour()Â function returns the current hour.
minute()Â function returns the current minute.
second()Â function returns the current seconds.
temp() function returns the current temperature of the ‘die’.